When I have started my first article series regarding How to unit testing Azure Blob Storage locally and GitHub few months back, I have never thought what comes next. I facing the same issue as I did with Azure Blob Storage, How can I unit test my Cosmos Db methods in .NET both locally and on GitHub with out necessarily having access to your Azure Portal or Access to Azure Cosmos Db account?
In this article series I will guidance you to this using .NET Core, so lets jump to it.
Pre-requestee
Docker desktop, in My case I use Windows OS 11, you might check the documentation for maxOS or Linux.
Introduction
To develop solution with Cosmos Db, you either need to have access to Azure Cosmos Db instance from Azure Cloud or having a local version of Cosmos Db called Azure Cosmos Db Emulator installed and running on your local environment.
In this part of this article I will focus on how to get Cosmos Db up and running on your local environment using docker.
Lets dot it.
Step1: Pull Azure Cosmos Db Emulator image
Start you command line and run following command to pull Azure Cosmos Db Emulator image:
docker pull mcr.microsoft.com/cosmosdb/linux/azure-cosmos-emulator
Resource from Microsoft.
Step2: Deploy Azure Cosmos Db Emulator
Now we have Azure Cosmos Db Emulator image downloaded, we need to deploy it using following command, in my case as mentioned, I do use Windows OS and Docker Desktop, but my docker is set to Linux.
docker run -p 8081:8081 -p 10251:10251 -p 10252:10252 -p 10253:10253 -p 10254:10254 -m 3g --cpus=2.0 --name=test-linux-emulator -e AZURE_COSMOS_EMULATOR_PARTITION_COUNT=10 -e AZURE_COSMOS_EMULATOR_ENABLE_DATA_PERSISTENCE=true -e AZURE_COSMOS_EMULATOR_IP_ADDRESS_OVERRIDE=127.0.0.1 -it mcr.microsoft.com/cosmosdb/linux/azure-cosmos-emulator
This will create an local instance of Cosmos Db.
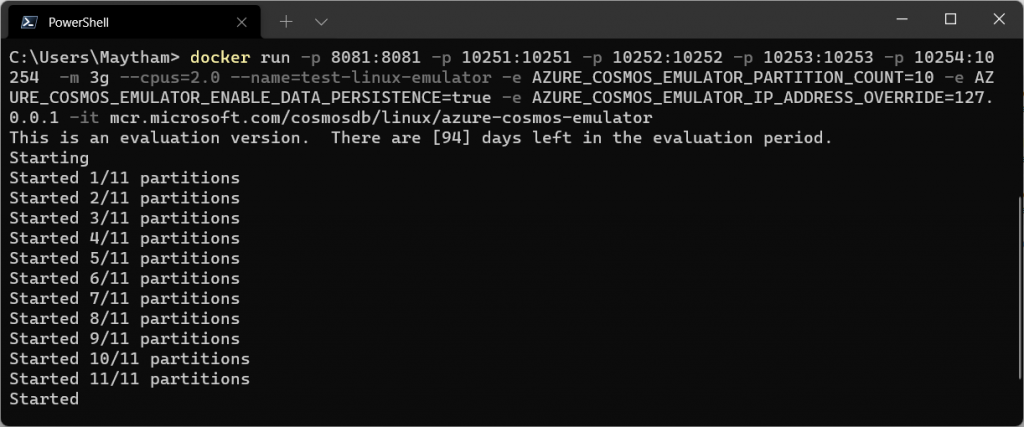
Step3: Brows with Azure Cosmos Db Emulator
The Azure Cosmos Db Emulator tools for view, browsing and manipulating Cosmos Db is an browser base tool. You should be able to access it while you Azure Cosmos Db Emulator docker instance is running.
- You need to open your browser
- Type
https://127.0.0.1:8081/_explorer/index.html
in your browser
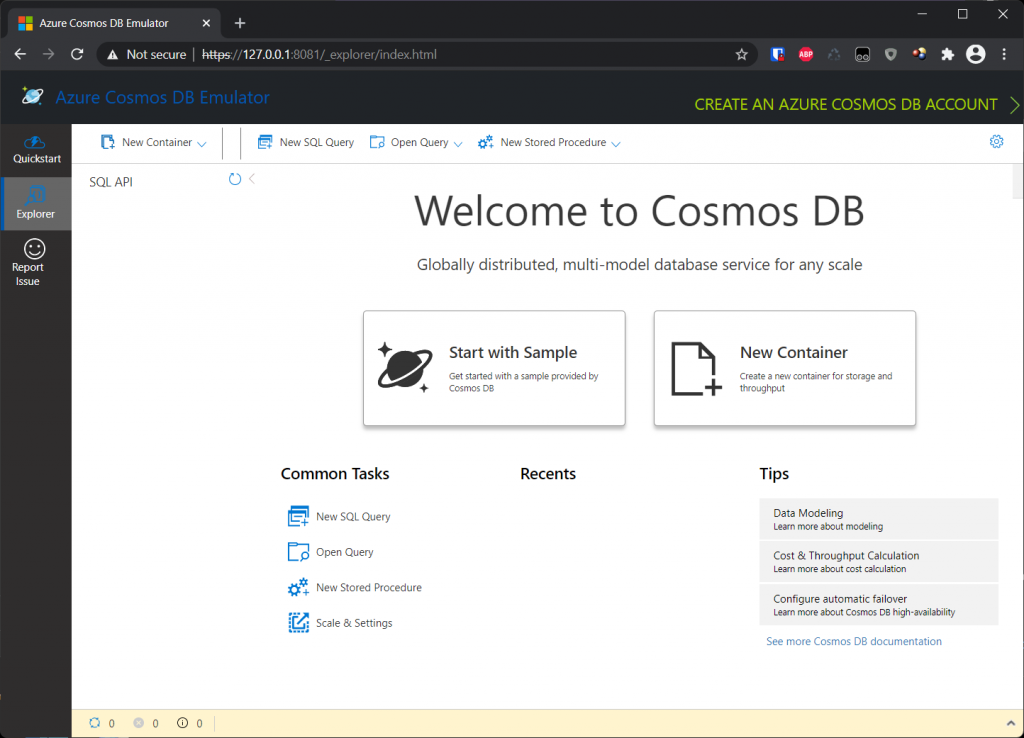
Here you should be able to Create Database, Container etc. as you are able to do the same from Azure Portal.
Step 4: Download Quick Start
If you now, click on QuickStart button, you will find 5 different platforms to try some basic code with:
- .NET
- .NET Core
- Java
- Node.js
- Python
In our case we will focus on .NET Core. Download it and try it out.
Step 5: Fix Certificate issue
in your quick start code, when you started the code, I guess you found an issue with it. That is correct, an issue with SSL connection like:
---> System.Net.Http.HttpRequestException: The SSL connection could not be established, see inner exception.
---> System.Security.Authentication.AuthenticationException: The remote certificate is invalid according to the validation procedure.
.... etc
You will find a CosmosClient class that has a default CosmosClientOptions in your quick start code. You need to added the following CosmosClientOptions:
CosmosClientOptions cosmosClientOptions = new CosmosClientOptions()
{
HttpClientFactory = () =>
{
HttpMessageHandler httpMessageHandler = new HttpClientHandler()
{
ServerCertificateCustomValidationCallback = HttpClientHandler.DangerousAcceptAnyServerCertificateValidator
};
return new HttpClient(httpMessageHandler);
},
ConnectionMode = ConnectionMode.Gateway
};
CosmosClient client = new CosmosClient(endpoint, authKey, cosmosClientOptions);
You will face issue with HttpClientFactory is not getting resolved. This requires you to update Microsoft.Azure.Cosmos
package to 3.23.0 as shown below.
<ItemGroup>
<PackageReference Include="Microsoft.Azure.Cosmos" Version="3.23.0" />
</ItemGroup>
When done, try to run your code, and it should works now. Congrats, so far so good.
Documentation reference for above.
Conclusion
So in this article we have learned how to pull Azure Cosmos Db Emulator image, deploying Azure Cosmos Db Emulator and start browser tool for Azure Cosmos Db Emulator.
You have also learned to download quick start with initial code for Cosmos Db and also how to fix certificate issue for testing. But for my next article, I will cover and focus on unit testing example and try to get it up and running with GitHub actions CI as well.
As you can see Microsoft are doing great job creating the required tools we need as developer to get the job done.
HttpClientHandler.DangerousAcceptAnyServerCertificateValidator is not the efficient way. It would be geat to know how to do the proper configuration of the local certificate
Somehow I agree with the point of your intention to work with the production database but remember when you test stuff in your playground locally I assume you are not working with real and sensitive data. And therefore you need local certificate, that said if you really need that let me know I will consider looking at it in future update.