I have recently met a nice challenge of moving a frontend repository let’s call it (A) with its commit history to an existing backend git repository let’s call (B).
At the begging of this challenge, I was thinking to clone repository A and copying it into a subfolder in repository B. This would work but without moving the commit history. Now in my case, I wanted to keep track of my commit history from (A).
In addition, the A repo has main and develop branches, as well as the B repo. So we have 2 branches with commits history.
Enough talk. Let’s jump to it.
Make sure, to always backup of your repository before you major change like this, just in case things go bad.
Before going and doing anything in my production repository, I created 2 sample repositories in GitHub, one called backend and the other the frontend.
I create a migration folder and I cloned both empty repositories in my migration folder:
git clone https://github.com/maythamfahmi/backend
git clone https://github.com/maythamfahmi/frontend
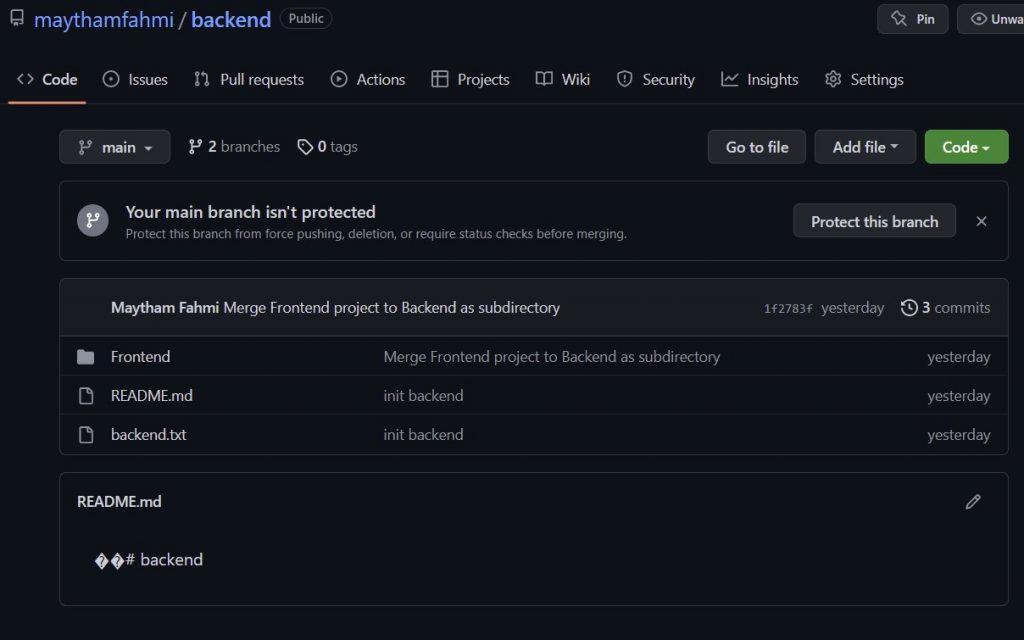
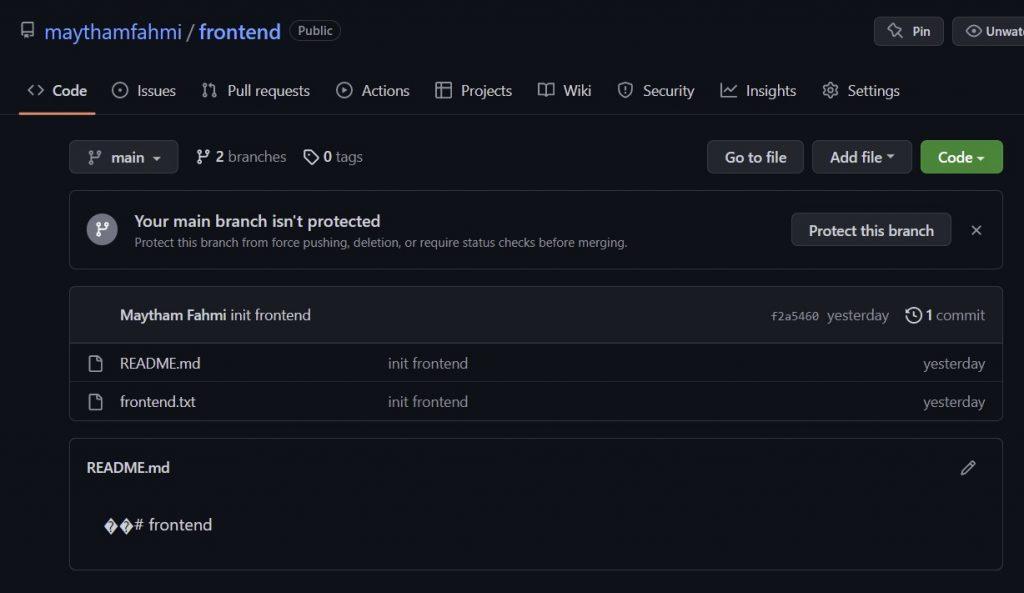
for each repository, I have created 2 branches, a main and develop branch. Besides that, I created just some random content in my backend and frontend so I have a kind of commit history for both branches.
Now the main logic for achieving the goal of the repository move is following the git script snippet with an inline explanation:
# from backend repo (B) folder
# I add the frontend repository as remote (remember to check it to main) and give it a name frontend
git remote add -f frontend C:\Workspace\RepoMigration\frontend\
# I merge the commit history of main to my current repo B
git merge -s ours --allow-unrelated-histories --no-commit frontend/main
# I read the everything from out frontend repo and copy it over to a Frondend folder inside my current repo
git read-tree --prefix=Frontend/ -u frontend/main
# Now I commit all changes made with a note
git commit -m "Merge Frontend project to Backend as subdirectory"
# Final step, I remove the remote repository
git pull -s subtree frontend main
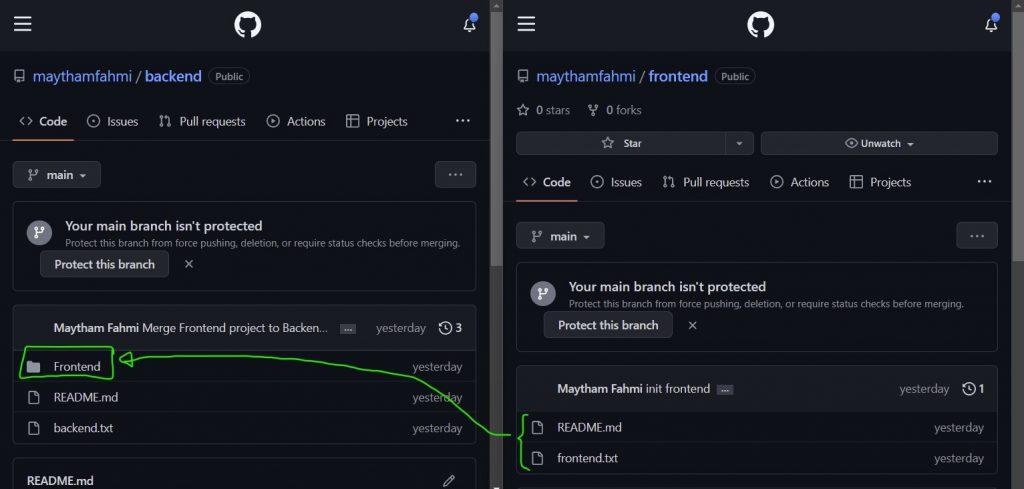
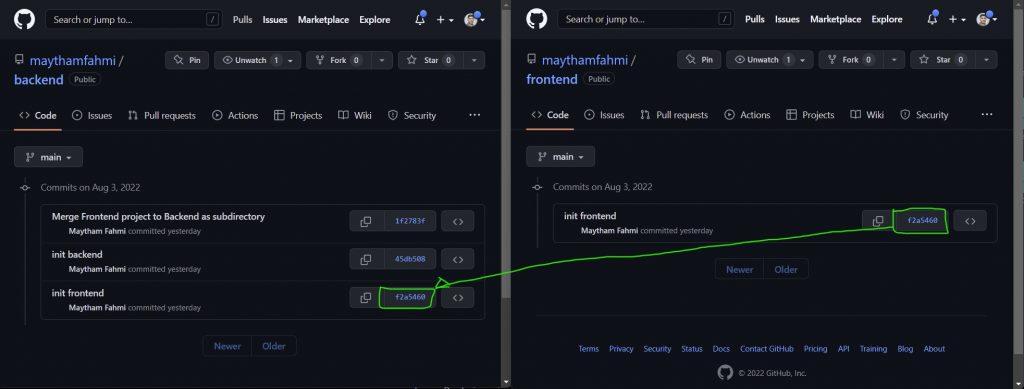
As you can see what we have achieved by using the above-mentioned git snippet, is we have moved the files of the frontend repository to a Frontend folder inside the backend repository. Furthermore, we have also moved the commit history from the frontend repository to the backend repository. as you can see in the Commit history image.
I have made a script of all steps I have explained in my article and put all commands together to demonstrate how I did it. I would suggest not using push until you validate all things play well on the local repository and then if all goes well, push it.
git clone https://github.com/maythamfahmi/frontend
git clone https://github.com/maythamfahmi/backend
cd frontend
echo "# frontend" >> README.md
echo "# init frontend" >> frontend.txt
git add .
git commit -m "init frontend"
git push
git checkout -b "develop"
echo "# frontend develop" >> README.md
echo "# some code for frontend development" >> frontend-development.txt
git add .
git commit -m "develop branch"
git push --set-upstream origin develop
git checkout main
cd..
cd backend
echo "# backend" >> README.md
echo "# init backend" >> backend.txt
git add .
git commit -m "init backend"
git push
git checkout -b "develop"
echo "# backend develop" >> README.md
echo "# some code for backend development" >> backend-development.txt
git add .
git commit -m "develop branch"
git push --set-upstream origin develop
git checkout main
git remote add -f frontend C:\Workspace\RepoMigration\frontend\
git merge -s ours --allow-unrelated-histories --no-commit frontend/main
git read-tree --prefix=Frontend/ -u frontend/main
git commit -m "Merge Frontend project to Backend as subdirectory"
git pull -s subtree frontend main
git push
git checkout develop
git merge -s ours --allow-unrelated-histories --no-commit frontend/develop
git read-tree --prefix=Frontend/ -u frontend/develop
git commit -m "Merge Frontend project to Backend as subdirectory"
git pull -s subtree frontend develop
git push
git checkout main
That is it. with a few lines of git magic, now we have moved the frontend repository in a subfolder to the backend repository including the commit history for both the main and develop branches.
Conclusion
As we have seen, I did this solution on GitHub using the git command line using git bash on Windows 11. The same command should be able to do the job for other version control tools like Azure DevOps, Bitbucket, GitLab, and others on other environments as well.