Merging pdf in C# is not straight forward task without using 3rd party library.
I have seen and used many libraries and NuGet packages that are free but have a limitation or are free only for private use. Now what to do if you are a little business and want to use a library without limitation for your business?
Why can not pdf file PDF merged out of the box with out using library? Pdf files behind the scenes has a strict file format that describes different aspects like Pdf version, the objects contained in the file and where to find them. You can learn more about it reading the basic about pdf and more insigtht about pdf.
As far as I have researched, I found an Open Source library called PdfClown for Java and .Net. For .net they have a NuGet package. The nice thing about PdfClown, it is FREE without limitation (donate if you like) and you can use it for your business as well. The library has a lot of features. In this article, I will show examples of how to merge 2 or more pdf documents into one document.
I supply my example that takes a folder with multiple pdf files and saves a merged version to the same or another folder.
The code is self-explaining, the key point here is using SerializationModeEnum.Incremental
:
public static void MergePdf(string srcPath, string destFile)
{
var list = Directory.GetFiles(Path.GetFullPath(srcPath));
if (string.IsNullOrWhiteSpace(srcPath) || string.IsNullOrWhiteSpace(destFile) || list.Length <= 1)
return;
var files = list.Select(File.ReadAllBytes).ToList();
using (var dest = new org.pdfclown.files.File(new org.pdfclown.bytes.Buffer(files[0])))
{
var document = dest.Document;
var builder = new org.pdfclown.tools.PageManager(document);
foreach (var file in files.Skip(1))
{
using (var src = new org.pdfclown.files.File(new org.pdfclown.bytes.Buffer(file)))
{ builder.Add(src.Document); }
}
dest.Save(destFile, SerializationModeEnum.Incremental);
}
}
To test it
var srcPath = @"C:\temp\pdf\input";
var destFile = @"c:\temp\pdf\output\merged.pdf";
MergePdf(srcPath, destFile);
Input examples
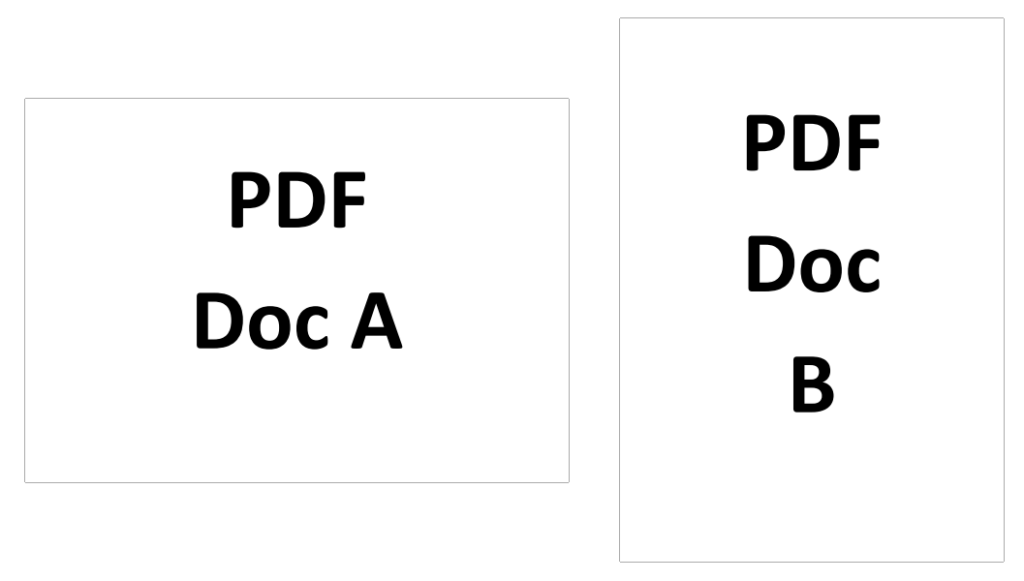
PDF doc A and PDF doc B
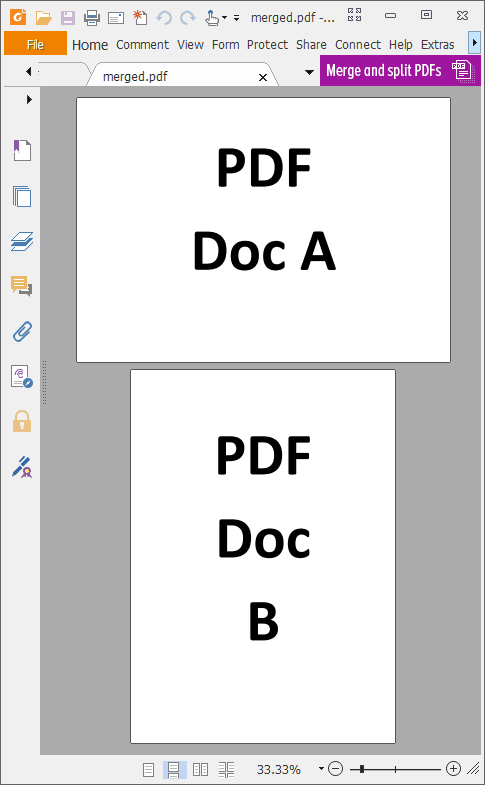
Source code GitHub.
Hi Maytham Fahmi,
I would like to try the source code. The link you supplied is not valid.
Can you send me the working link/page?
Thanks.
Tjetram
Thank you for reaching out. I have updated the link in the article and here is the link as well:
https://github.com/maythamfahmi/BlogExamples/tree/main/Examples/ImgConverter